Generating Access Token for Google Cloud Platform API Access using Service Accounts

In order to access online Google Cloud Platform APIs via Servers, we need an access token for authorization. In this story , we will see how to generate the same.
Objective:
- Generate access token for accessing the google cloud platform APIs.
Steps:
- Create a service account with the required role.
- Generate the access token using the scope and service account created above.
For our understanding , we would consider a use case where a custom model is uploaded on the Google Cloud Platform -> AI Platform and inference needs to be made using the REST API.
Step 1:
- First we need to identify the permissions that we need for our use case. Since we want to perform predictions on out custom uploaded model, we would see that that the permissions that we need are
ml.models.predict
andml.versions.predict
. Please refer https://cloud.google.com/ai-platform/prediction/docs/reference/rest/v1/projects/predict for more details. - We can also find the OAuth Scope in the above link as “https://www.googleapis.com/auth/cloud-platform”. We would need the same during access token generation.
- Since these permissions are not available as direct roles , we would need to create a custom role and associate these permissions to the role.
- Let’s open Google Cloud Console i.e. https://console.cloud.google.com/
- Goto Home->IAM & Admin -> Roles

- Click on “Create Role” to proceed.

- Please fill in the necessary details for the newly created Role. Once you have provided the details, click on Add Permissions.

- Once you have selected the required 2 permissions for our use case , click on “CREATE” button to proceed.

- You will observe that the required role is created.
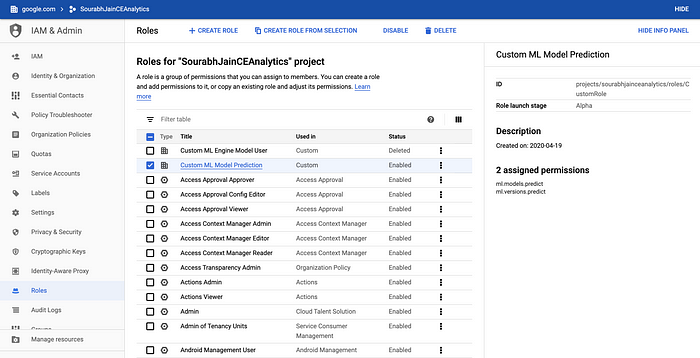
- Now we will create a service account and associate this role to the service account.
- Goto Home-> IAM & Admin -> Service Account

- Click on “CREATE SERVICE ACCOUNT” to create a new service account.
- Provide the service account details and click on CREATE.

- Select the custom role that has been create and click on “CONTINUE”.

- Click on “+ CREATE KEY”. Set the Key Type as “JSON”. Click on “CREATE”. This will download the JSON file to the local machine.
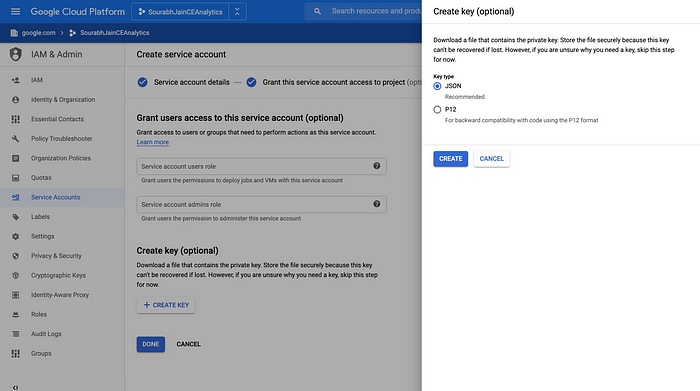
- Keep the service account file safe and don’t share with unauthorized users.
- This completes the Step 1.
Step 2 :
- Now we need to generate the access token that is required for our API authorization.
- Please copy the below 2 scripts to the folder where the service account json file downloaded in step 1 is kept.
#!/bin/bash
set -euo pipefail
base64var() {
printf "$1" | base64stream
}
base64stream() {
base64 | tr '/+' '_-' | tr -d '=\n'
}
key_json_file="$1"
scope="$2"
valid_for_sec="${3:-3600}"
private_key=$(jq -r .private_key $key_json_file)
sa_email=$(jq -r .client_email $key_json_file)
header='{"alg":"RS256","typ":"JWT"}'
claim=$(cat <<EOF | jq -c
{
"iss": "$sa_email",
"scope": "$scope",
"aud": "https://www.googleapis.com/oauth2/v4/token",
"exp": $(($(date +%s) + $valid_for_sec)),
"iat": $(date +%s)
}
EOF
)
request_body="$(base64var "$header").$(base64var "$claim")"
signature=$(openssl dgst -sha256 -sign <(echo "$private_key") <(printf "$request_body") | base64stream)
printf "$request_body.$signature"
The above script “jwttoken.sh” script accepts the service account json file & auth scope and returns JWT Token. The script uses the private key from the service account and generates JWT Token using openssl.
- Using the above script , we will now generate the access token.
#!/bin/bash
set -euo pipefail
key_json_file="$1"
scope="$2"
jwt_token=$(./jwttoken.sh "$key_json_file" "$scope")
curl -s -X POST https://www.googleapis.com/oauth2/v4/token \
--data-urlencode 'grant_type=urn:ietf:params:oauth:grant-type:jwt-bearer' \
--data-urlencode "assertion=$jwt_token" \
| jq -r .access_token
- The above script “accesstoken.sh” accepts the service account JSON file and Auth Scope. It calls “jwttotken.sh” file and uses the generated JWT Token to invoke the OAuth API and generate the Access Token.
- Please provide the execute privileges to both the scripts “chmod +x *.sh”.

- Execute the script and you will get the access token.

- The access token is generated.
- Please leave a comment if any query and I will be happy to help.
- Code Reference taken from below link : https://gist.github.com/ryu1kn/c76aed0af8728f659730d9c26c9ee0ed